
Java is one of the most popular programming languages out there. Released in 1995 and still widely used today, Java has many applications, including software development, mobile applications, and large systems development. Knowing Java opens a lot of possibilities for you as a developer.
About the Trainer
Introduction
- Name: John Smith
- Professional Title: Senior Software Engineer
- Education: Master’s in Computer Science, XYZ University
- Certifications:
- Java Certified Programmer
- Oracle Certified Professional (OCP-JD)
- Expertise:
- Over 15 years of experience in software development and architecture
- Proficient in full-stack development with expertise in Java and web application development
- Industry Experience:
- Worked with leading tech companies, contributing to the development of scalable and secure applications
- Extensive experience in designing and implementing cloud-based solutions for diverse industries
- Training Experience:
- Conducted numerous training sessions on cloud architecture, software development best practices, and agile methodologies
- Received positive feedback for clear communication and hands-on, practical training approach
- Skills:
- Strong communication and presentation skills
- Ability to convey complex technical concepts in an understandable manner
- Passionate about fostering a collaborative and engaging learning environment
Checklist
Skill level
Beginner to Advanced
Time to complete
25 to 30 hrs online mode: LIVE session, Java SE Programmer
Key take aways
e-book and certification of completion
Prerequisites
None – anyone who read or write English, can takeup this course
Offer
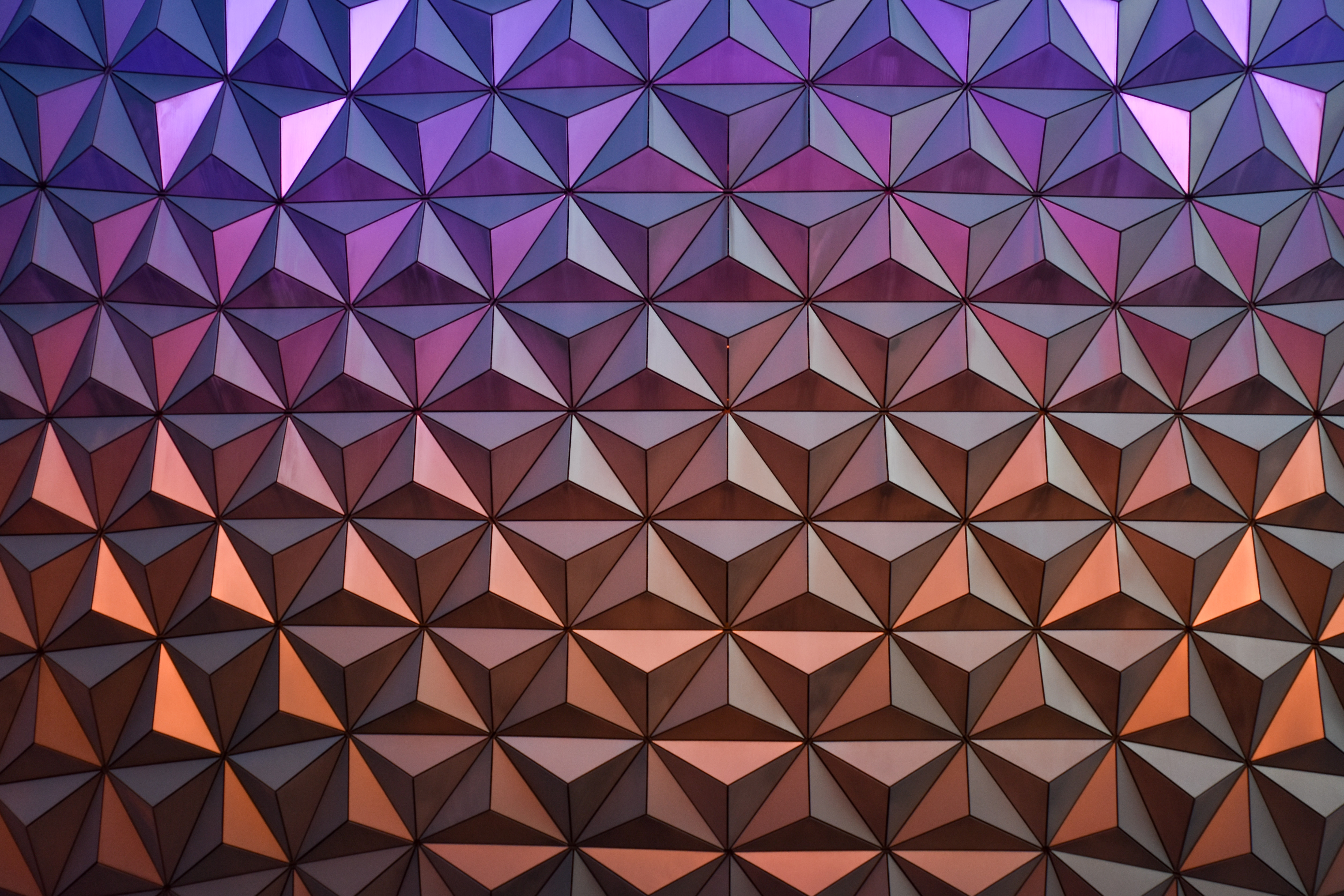
10% Off on
EARLY BIRD OFFER
About this course
A comprehensive Java programming course offers a systematic exploration of the language, covering foundational concepts, syntax, and object-oriented programming. Participants progress through advanced topics like multithreading, networking, and database connectivity. The course delves into user interface development with JavaFX, Swing, and JavaServer Pages. Web development is introduced using Servlets, JSP, and the Spring Framework. Practical application is emphasized through project work, ensuring hands-on experience. The course also covers advanced Java features and concurrency utilities, providing participants with the skills to develop practical, real-world applications.
Syllabus
Module 1: Introduction to Java
- Introduction to Programming:
- Basics of programming logic
- Understanding algorithms
- Introduction to Java:
- History and evolution of Java
- Java development environment setup
Module 2: Java Basics
- Java Syntax and Structure:
- Variables, data types, and operators
- Control flow statements (if, switch, loops)
- Object-Oriented Programming (OOP) Concepts:
- Classes and objects
- Encapsulation, inheritance, and polymorphism
- Methods and Functions:
- Defining and calling methods
- Method overloading and overriding
Module 3: Core Java Programming
- Arrays and Collections:
- Working with arrays
- Introduction to Java Collections Framework
- Exception Handling:
- Handling exceptions with try-catch blocks
- Creating custom exceptions
- File Handling:
- Reading and writing files in Java
- Working with streams
Module 4: Advanced Java Programming
- Multithreading:
- Introduction to threads
- Synchronization and thread safety
- Networking in Java:
- Socket programming
- URL and HTTP connections
- Java Database Connectivity (JDBC):
- Connecting to databases
- Executing SQL queries in Java
Module 5: Java User Interface (UI)
- Introduction to JavaFX:
- Building graphical user interfaces
- Event handling in JavaFX
- Swing and AWT:
- Creating desktop applications
- GUI components and layouts
Module 6: Web Development with Java
- Servlets:
- Introduction to Java Servlets
- Handling HTTP requests and responses
- JavaServer Pages (JSP):
- Creating dynamic web pages
- Integrating Java code into HTML
- Introduction to Spring Framework:
- Overview of the Spring framework
- Dependency injection and inversion of control
Module 7: Java Persistence and ORM
- Introduction to Hibernate:
- Object-Relational Mapping (ORM)
- Hibernate configuration and mapping
- Spring Data JPA:
- Working with Java Persistence API (JPA) in Spring
- Repository interfaces and CRUD operations
Module 8: Web Services in Java
- Introduction to RESTful Web Services:
- Design principles of REST
- Creating RESTful services in Java
- SOAP Web Services with JAX-WS:
- Building and consuming SOAP services
- Java API for XML Web Services (JAX-WS)
Module 9: Testing and Debugging
- Unit Testing with JUnit:
- Writing and executing JUnit tests
- Test-driven development (TDD)
- Debugging Java Applications:
- Using debugging tools and techniques
Module 10: Project Work and Real-world Applications
- Building a Java Project:
- Applying learned concepts to a project
- Best practices and code optimization
- Code Review and Collaboration:
- Collaborative coding using version control (Git)
- Code review practices
Module 11: Advanced Topics
- Introduction to Java 9 and Beyond:
- New features and enhancements in recent Java versions
- Concurrency Utilities:
- Exploring java.util.concurrent package